jQuery cool Password strength verification plugin
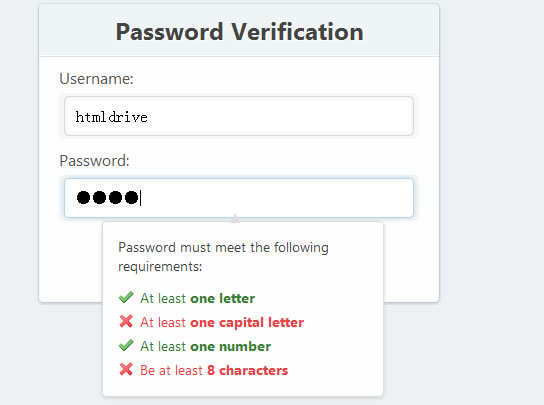
Many sites that require login credentials enforce a security setting often referred to as password complexity requirements. These requirements ensure that user passwords are sufficiently strong and cannot be easily broken.
What constitutes a strong password? Well, that depends on who you ask. However, traditional factors that contribute to a password’s strength include it’s length, complexity, and unpredictability. To ensure password strength, many sites require user passwords to be alphanumeric in addition to being a certain length.
In this tutorial, we’ll construct a form that gives the user live feedback as to whether their password has sufficiently met the complexity requirements we will establish.
Step 1: Starter HTML
First we want to get our basic HTML starter code. We’ll use the following:
<!DOCTYPE html> <html> <head> <title>Password Verification</title> </head> <body> <div id="container"> <-- Form HTML Here --> </div> </body> </html>
Step 2: Form HTML
Now let’s add the markup that will be used for our form. We will wrap our form elements in list items for better structure and organization.
<h1>Password Verification</h1> <form> <ul> <li> <label for="username">Username:</label> <span><input id="username" name="username" type="text" /></span> </li> <li> <label for="pswd">Password:</label> <span><input id="pswd" type="password" name="pswd" /></span> </li> <li> <button type="submit">Register</button> </li> </ul> </form>
Here’s an explanation of the code we used:
-
span
elements – these will be used for visual styling of our input elements (as you’ll see later on in the CSS) -
type="password"
– this is an HTML5 attribute for form elements. In supported browsers, the characters in this field will be replaced by black dots thus hiding the actual password on-screen.
Step 3: Password information box HTML
Now let’s add the HTML that will inform the user which complexity requirements are being met. This box will be hidden by default and only appear when the “password” field is in focus.
<div id="pswd_info"> <h4>Password must meet the following requirements:</h4> <ul> <li id="letter" class="invalid">At least <strong>one letter</strong></li> <li id="capital" class="invalid">At least <strong>one capital letter</strong></li> <li id="number" class="invalid">At least <strong>one number</strong></li> <li id="length" class="invalid">Be at least <strong>8 characters</strong></li> </ul> </div>
Read more:http://www.webdesignerdepot.com/2012/01/password-strength-verification-with-jquery/
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu