awsesome images gallery using css3 and jquery




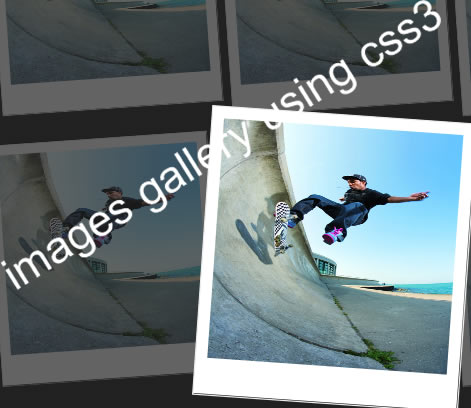
we will be creating a very simple but great looking css3 image gallery using jquery and css3. The image gallery will be tited to the side and on hover will move. Whenever you click on the image a lightbox will pop out.
The HTML Structure
For the image gallery I simply wrapped the gallery in a div and added the images. Every 4 image has two different classed assigned to them. For example the first 4 images has a class name of thumb-small and for the other 4 images they have a class name of thumb-bottom.
<a href="images/skate1.jpg" title="You can write anything in here"><img class="thumb-top" src="images/skate1.jpg" alt="skateboarder" title="skateboarder"/></a> <a href="images/skate1.jpg" title="You can write anything in here"><img class="thumb-top" src="images/skate1.jpg" alt="skateboarder" title="skateboarder"/></a> <a href="images/skate1.jpg" title="You can write anything in here"><img class="thumb-top" src="images/skate1.jpg" alt="skateboarder" title="skateboarder"/></a> <a href="images/skate1.jpg" title="You can write anything in here"><img class="thumb-top" src="images/skate1.jpg" alt="skateboarder" title="skateboarder"/></a> <a href="images/skate1.jpg" title="You can write anything in here"><img class="thumb-bottom" src="images/skate1.jpg" alt="skateboarder" title="skateboarder"/></a> <a href="images/skate1.jpg" title="You can write anything in here"><img class="thumb-bottom" src="images/skate1.jpg" alt="skateboarder" title="skateboarder"/></a> <a href="images/skate1.jpg" title="You can write anything in here"><img class="thumb-bottom" src="images/skate1.jpg" alt="skateboarder" title="skateboarder"/></a> <a href="images/skate1.jpg" title="You can write anything in here"><img class="thumb-bottom" src="images/skate1.jpg" alt="skateboarder" title="skateboarder"/></a>
The Css
For the Css you might wonder what -webkit, and -moz-transform is. Webkit is a Css3 tag that targets the safari web browser and moz-transform is a Css3 tag that targets the Mozilla Firefox web browser. In the css
- -webkit-transform: – Used to rotate the images
- -webkit-transition: – Used to zoom/ease in on the images
.thumb-top{ clear:none; padding:10px 10px 30px 10px; height:200px; width:200px; background:#fff; -moz-transform:rotate(-4deg); -webkit-transform:rotate(-4deg); -webkit-transition: -webkit-transform 0.1s ease-in; /* Tells webkit to make a transition of a transform */ border:1px solid #ececec; /* This border merges with the colour of the shadow in supporting browsers but in non-supporting browsers the border acts as the edge of the image */ margin-bottom:25px; } .thumb-bottom{ clear:none; padding:10px 10px 30px 10px; height:200px; width:200px; background:#fff; -moz-transform:rotate(-4deg); -webkit-transform:rotate(-4deg); -webkit-transition: -webkit-transform 0.1s ease-in; /* Tells webkit to make a transition of a transform */ border:1px solid #ececec; /* This border merges with the colour of the shadow in supporting browsers but in non-supporting browsers the border acts as the edge of the image */ margin-bottom:25px; } .thumb-top:hover{ z-index:100; position:relative; /* Make the z-index work in Safari */ -moz-transform:rotate(-8deg) scale(1.2); -webkit-transform:rotate(-8deg) scale(1.2); } .thumb-bottom:hover{ z-index:100; position:relative; /* Make the z-index work in Safari */ -moz-transform:rotate(4deg) scale(1.2); -webkit-transform:rotate(4deg) scale(1.2); } #gallery { margin-top:20px; }
Inserting and activating jquery plugins
First things first when activating a jquery plugin is to simply make sure we call in the jquery script. Insert the code below into the head section of your document. The code below calls in jquery, then the two jquery plugins, and finally the plugin Css.
<link rel="stylesheet" type="text/css" href="css/jquery.lightbox-0.5.css" media="screen" /> <script type="text/javascript" src="js/jquery.lightbox-0.5.js"></script> <script type="text/javascript" src="js/jquery-1.3.2.min.js"></script> <script type="text/javascript" src="js/jquery.lightbox-0.5.js"></script>
Now we want to add the script below to the body of our HTML document right before the end of the body tag.
<script type="text/javascript"> $(document).ready(function(){ $(".thumb-top,.thumb-bottom").fadeTo("slow", 0.3); $(".thumb-top,.thumb-bottom").hover(function(){ $(this).fadeTo("slow", 1.0); },function(){ $(this).fadeTo("slow", 0.3); }); }); $(function() { $('#gallery a').lightBox(); }); </script>
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu