cool Typing Game with jQuery
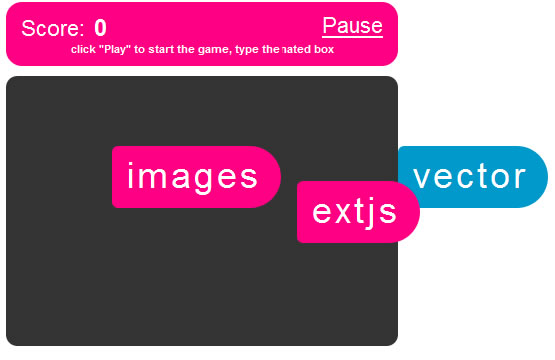
Continue from my previous post, about detecting character in jquery, now try to some more sophisticated example, this game inspired by an old shark typing game, which is created by pop cap game, but it seems not available anymore.
The rules of the this game is really simple, you have to type the word that suit on text inside the animated box, finish a word you got some point, and there will be another word to type.
The HTML
The html element contain 2 main element, first is div for score board and play control, and second is the div that have all the animated box.
<div id="toolbar"> <div id="boxscore"> <span>Score:</span> <span id="score">0</span> </div> <div id="boxcontrol"> <a href="" id="btnplay">Play</a> </div> </div> <div id="container"> <div class="animatedbox"> <span class="match"></span><span class="unmatch"></span> </div> <div class="animatedbox"> <span class="match"></span><span class="unmatch"></span> </div> </div>
The JQuery
First we have to declare all the words that we want to display, we can use javascript array, and then we can pick one on random.
var arrstring = new Array("images", "wallpaper", "photos", "vector", "designer", "wordpress", "jquery", "extjs", "scripting", "blogging", "search", "tagging", "digital", "javascript", "server", "hosting", "social", "twitter", "graphic", "photoshop", "netbeans", "mysql", "apache", "iphone", "mobile", "android", "framework", "usability", "optimization", "interface", "developer");
Now for the JQuery main code. Line [001 - 003]: the random function from admixweb that is very handy to use, I use this in some of previous post. Line [024 - 040]: function when “play” link click, it used for pausing the game too. Line [049 - 086]: funtion startPlay(), the main function to animate the div that contain random string. Line [089 - 129]: implementation of keypress event. In this function if the input character match on the text inside a span, it will moved to another span, but user seen this as change the color
function randomFromTo(from, to){ return Math.floor(Math.random() * (to - from + 1) + from); } var score = 0; $(document).ready(function() { var children = $("#container").children(); var child = $("#container div:first-child"); var currentEl; var currentElPress; var win_width = $(window).width(); var text_move_px = 500; var box_left = (win_width / 2) - (text_move_px / 2); var playGame; var stop; $(".animatedbox").css("left", box_left+"px"); $("#btnplay").click(function() { if ($(this).text() == "Play") { startPlay(); playGame = setInterval(startPlay, 23000); $(this).text("Pause"); } else if ($(this).text() == "Pause") { stop = true; if ($("#container").find(".current").length == 0) { $(this).text("Play"); } else { $(this).text("wait a moment"); } clearInterval(playGame); } return false; }); var con_height = $("#container").height(); var con_pos = $("#container").position(); var min_top = con_pos.top; // 56 = animated box top & bottom padding + font size var max_top = min_top + con_height - 56; function startPlay() { child = $("#container div:first-child"); child.addClass("current"); currentEl = $(".current"); for (i=0; i<children.length; i++) { var delaytime = i * 3500; setTimeout(function() { randomIndex = randomFromTo(0, arrstring.length - 1); randomTop = randomFromTo(min_top, max_top); child.animate({"top": randomTop+"px"}, 'slow'); child.find(".match").text(""); child.find(".unmatch").text(arrstring[randomIndex]); child.show(); child.animate({ left: "+="+text_move_px }, 8000, function() { currentEl.removeClass("current"); currentEl.fadeOut('fast'); currentEl.animate({ left: box_left+"px" }, 'fast'); if (currentEl.attr("id") == "last") { child.addClass("current"); currentEl = $(".current"); if (stop) { $("#btnplay").text("Play"); } } else { currentEl.next().addClass("current"); currentEl = currentEl.next(); } }); child = child.next(); }, delaytime); } } // on ie $(window).keypress() won't work $(document).keypress(function(event) { currentElPress = $(".current"); var matchSpan = currentElPress.find(".match"); var unmatchSpan = currentElPress.find(".unmatch"); var unmatchText = unmatchSpan.text(); var inputChar; if ( $.browser.msie || $.browser.opera ) { inputChar = String.fromCharCode(event.which); } else { inputChar = String.fromCharCode(event.charCode); } if (inputChar == unmatchText.charAt(0)) { unmatchSpan.text(unmatchText.replace(inputChar, "")); matchSpan.append(inputChar); if (unmatchText.length == 1) { currentElPress.stop().effect("explode", 500); currentElPress.animate({ left: box_left+"px" }, 'fast'); if (currentElPress.attr("id") == "last" && stop) { $("#btnplay").text("Play"); } currentElPress.removeClass("current"); currentElPress = currentElPress.next(); currentElPress.addClass("current"); currentEl = currentElPress; score += 50; $("#score").text(score).effect("highlight", { color: '#000000' }, 1000); } else { score += 10; $("#score").text(score); } } }); });
The CSS
* { font-family: Arial; } #container { background: #333; width: 500px; height: 230px; margin: 0 auto; -webkit-border-radius: 0.7em; -moz-border-radius: 0.7em; border-radius: 0.7em; padding: 20px 0; } .animatedbox { background: #ff0084; position: absolute; padding: 8px 20px 12px 15px; font-size: 36px; color: #ffffff; -webkit-border-radius: 0.5em 2.5em 2.5em 0.5em; -moz-border-radius: 0.5em 2.5em 2.5em 0.5em; border-radius: 0.5em 2.5em 2.5em 0.5em; left: 500px; letter-spacing: 3px; display: none; } .match { color: #000000; } .current { background: #0099cc; } #toolbar { background: #ff0084; -webkit-border-radius: 1.0em; -moz-border-radius: 1.0em; border-radius: 1.0em; width: 500px; padding: 10px 0 10px 0; margin: 0 auto; text-align: center; margin-bottom: 10px; margin-top: 10px; } #boxscore { float: left; font-size: 22px; margin-left: 15px; color: #ffffff; } #score { font-weight: bold; font-size: 24px; padding: 0 3px; } #boxcontrol { float: right; margin-right: 15px; } #boxcontrol a { font-size: 22px; color: #ffffff; } .clear { margin-top: 5px; font-size: 11px; font-weight: bold; color: #ffffff; clear: both; }
The article source:http://superdit.com/2011/03/04/jquery-typing-game/
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu