jQuery Text Cloud effect
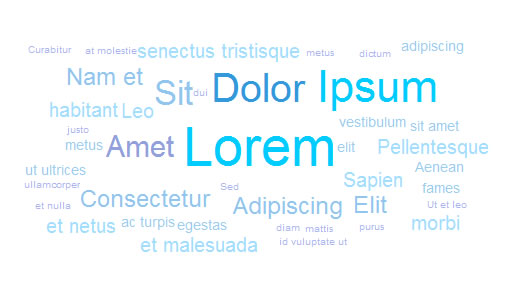
jQCloud is a jQuery plugin that builds neat and pure HTML + CSS word clouds and tag clouds that are actually shaped like a cloud
Installation
Installing jQCloud is extremely simple:
- Make sure to import the jQuery library in your project.
- Download the jQCloud files. Place jqcloud-0.2.10.js (or the minified version jqcloud-0.2.10.min.js) and jqcloud.css somewhere in your project and import both of them in your HTML code.
You can easily substitute jqcloud.css with a custom CSS stylesheet following the guidelines explained later.
Usage
Once you imported the .js and .css files, drawing a cloud is as simple as this:
<!DOCTYPE html> <html> <head> <title>jQCloud Example</title> <link rel="stylesheet" type="text/css" href="jqcloud.css" /> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.4/jquery.min.js"></script> <script type="text/javascript" src="jqcloud-0.2.10.js"></script> <script type="text/javascript"> /*! * Create an array of objects to be passed to jQCloud, each representing a word in the cloud */ var word_list = [ {text: "Lorem", weight: 15}, {text: "Ipsum", weight: 9, url: "http://jquery.com/", title: "I can haz URL"}, {text: "Dolor", weight: 6}, {text: "Sit", weight: 7}, {text: "Amet", weight: 5} // ...other words ]; $(document).ready(function() { // Call jQCloud on a jQuery object passing the word list as the first argument. Chainability of methods is maintained. $("#example").jQCloud(word_list); }); </script> </head> <body> <!-- You must explicitly specify the dimensions of the container element --> <div id="example" style="width: 550px; height: 350px; position: relative;"></div> </body> </html>
Word Attributes
For each word, you need to specify the following mandatory attributes:
- text (string): the word(s) text
- weight (number): a number (integer or float) defining the relative importance of the word (such as the number of occurrencies, etc.). The range of values is arbitrary, as they will be linearly mapped to a discrete scale from 1 to 10.
You can also specify the following optional attributes for each word:
-
url (string): if specifyed, the word will be wrapped in a HTML link (
<a>
tag) and the URL used as itshref
attribute. -
title (string): an HTML title for the
<span>
that will contain the word(s) -
callback (callable): a function to be called after this word is rendered. Within the function,
this
refers to the jQuery object for the<span>
containing the word. - customClass (string): an additional HTML class for the word
- dataAttributes (object): keys and values to be used as HTML5 data attributes for the word (e.g. {confirm: "Are you sure?", remote: true})
- handlers (object): an object specifying event handlers that will bind to the word (e.g.: {click: function() { alert("it works!"); } })
Options:
Since version 0.2.0, jQCloud accepts an object containing configuration options as the second argument:
Read more:https://github.com/DukeLeNoir/jQCloud
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu