Photo Slider with jQuery




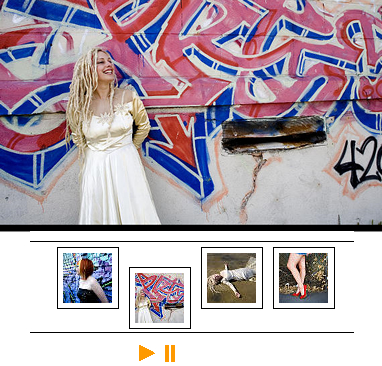
Step 1 - Pick Your Photos
First we need to start off with some images. You'll want these images to be thumbnails that also have a larger view available. In this example I'm using a thumbnail size of 50x50 and a full view of 600x400. You can have any size for the "full view" but the thumbnails must all be the same size. We'll start with 4 thumbnails.
Step 2 - Import Your Images
Now we need to tell our photo slider which images we want to use. Here we can either set them directly using the bucket hash or we can give it an array of image ids.
in the <head> tag
<link rel="stylesheet" type="text/css" media="screen" href="photoslider.css" />
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript" src="photoslider.js"></script>
in the <body> tag
<div class="photoslider" id="default"></div>
<script type="text/javascript">
$(document).ready(function(){
//change the 'baseURL' to reflect the host and or path to your images
FOTO.Slider.baseURL = 'http://example.com/path/';
//set images by filling our bucket directly
FOTO.Slider.bucket = {
'default': {
0: {'thumb': 't_0.jpg', 'main': '0.jpg', 'caption': 'Opie'},
1: {'thumb': 't_1.jpg', 'main': '1.jpg'},
2: {'thumb': 't_2.jpg', 'main': '2.jpg', 'caption': 'Trash the Dress'},
3: {'thumb': 't_3.jpg', 'main': '3.jpg'}
}
};
});
</script>
Step 3 - Load the Photo Slider
FOTO.Slider.reload('default');
Optional - Slideshow
If you want to you can add slideshow controls, along with automatically playing on load.
//enable the slideshow and build the controls FOTO.Slider.enableSlideshow('default'); //automatically play FOTO.Slider.play('default');
Optional - Preload Images
If you want the images to start pre-loading before a user clicks on them, run the preloadImages method.
FOTO.Slider.preloadImages('default');
Full JavaScript Code Used in Example
<script type="text/javascript"> $(document).ready(function(){ var ids = new Array(0,1,2,3); FOTO.Slider.importBucketFromIds('default',ids); FOTO.Slider.reload('default'); FOTO.Slider.preloadImages('default'); FOTO.Slider.enableSlideshow('default'); }); </script>
Notes
If you change the thumbnail width, you will need to modify the this.data[key]['thumbWidth'] in the reload function and set the number to the total width (width + border-left + border-right + margin-left + margin-right + padding-left + padding-right).
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu