Beautiful custom content scroller with jquery
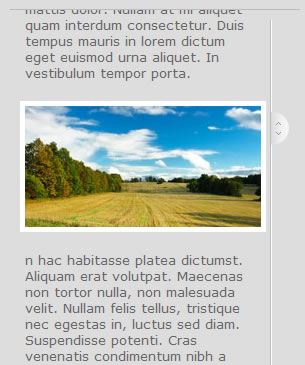
A custom content scroller created with jquery and css that supports mousewheel, scroll easing and has a fully customizable scrollbar.
The script utilizes jQuery UI and Brandon Aaron jquery mousewheel plugin. Very easy to configure and style with css.
The code
The css
body {margin:0; padding:0; background:#333;} #outer_container{margin:60px; width:260px; padding:0 10px; border-top:1px solid #666; border-bottom:1px solid #666;} #customScrollBox{position:relative; height:600px; overflow:hidden;} #customScrollBox .container{position:relative; width:240px; top:0; float:left;} #customScrollBox .content{clear:both;} #customScrollBox .content p{padding:10px 5px; margin:10px 0; color:#fff; font-family:Verdana, Geneva, sans-serif; font-size:13px;} #customScrollBox img{border:5px solid #fff;} #dragger_container{position:relative; width:0px; height:580px; float:left; margin:10px 0 0 10px; border-left:1px solid #000; border-right:1px solid #555;} #dragger{position:absolute; width:9px; height:40px; background:#999; margin-left:-5px; text-align:center; line-height:40px; color:#666; overflow:hidden; border-left:1px solid #ccc; border-right:1px solid #555;}
The jQuery scripts and plugins inside head tag (jquery and jquery UI are loaded straight from Google)
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4/jquery.min.js"></script> <script src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/jquery-ui.min.js"></script> <script type="text/javascript" src="jquery.easing.1.3.js"></script> <script type="text/javascript" src="jquery.mousewheel.min.js"></script>
The full javascript code is inserted in the end of the document, just before the closing body tag.
<script> $(window).load(function() { $customScrollBox=$("#customScrollBox"); $customScrollBox_container=$("#customScrollBox .container"); $customScrollBox_content=$("#customScrollBox .content"); $dragger_container=$("#dragger_container"); $dragger=$("#dragger"); visibleHeight=$customScrollBox.height(); if($customScrollBox_container.height()>visibleHeight){ //enable scrollbar if content is long totalContent=$customScrollBox_content.height(); minDraggerHeight=$dragger.height(); draggerContainerHeight=$dragger_container.height(); adjDraggerHeight=totalContent-((totalContent-visibleHeight)*1.3); //adjust dragger height analogous to content if(adjDraggerHeight>minDraggerHeight){ //minimum dragger height $dragger.css("height",adjDraggerHeight+"px").css("line-height",adjDraggerHeight+"px"); } else { $dragger.css("height",minDraggerHeight+"px").css("line-height",minDraggerHeight+"px"); } if(adjDraggerHeight<draggerContainerHeight){ //maximum dragger height $dragger.css("height",adjDraggerHeight+"px").css("line-height",adjDraggerHeight+"px"); } else { $dragger.css("height",draggerContainerHeight-10+"px").css("line-height",draggerContainerHeight-10+"px"); } animSpeed=400; //animation speed easeType="easeOutCirc"; //easing type bottomSpace=1.05; //bottom scrolling space targY=0; draggerHeight=$dragger.height(); $dragger.draggable({ axis: "y", containment: "parent", drag: function(event, ui) { Scroll(); }, stop: function(event, ui) { DraggerOut(); } }); //scrollbar click $dragger_container.click(function(e) { $this=$(this); $this.css("background-color","#555"); var mouseCoord=(e.pageY - $this.offset().top); var targetPos=mouseCoord+$dragger.height(); if(targetPos<$dragger_container.height()){ $dragger.css("top",mouseCoord); Scroll(); } else { $dragger.css("top",$dragger_container.height()-$dragger.height()); Scroll(); } }); //mousewheel $(function($) { $customScrollBox.bind("mousewheel", function(event, delta) { vel = Math.abs(delta*10); $dragger.css("top", $dragger.position().top-(delta*vel)); Scroll(); if($dragger.position().top<0){ $dragger.css("top", 0); $customScrollBox_container.stop(); Scroll(); } if($dragger.position().top>$dragger_container.height()-$dragger.height()){ $dragger.css("top", $dragger_container.height()-$dragger.height()); $customScrollBox_container.stop(); Scroll(); } return false; }); }); //scroll function Scroll(){ var scrollAmount=(totalContent-(visibleHeight/bottomSpace))/(draggerContainerHeight-draggerHeight); var draggerY=$dragger.position().top; targY=-draggerY*scrollAmount; var thePos=$customScrollBox_container.position().top-targY; $customScrollBox_container.stop().animate({top: "-="+thePos}, animSpeed, easeType); //with easing //$customScrollBox_container.css("top",$customScrollBox_container.position().top-thePos+"px"); //no easing } $dragger.mouseup(function(){ DraggerOut(); }).mousedown(function(){ DraggerOver(); }); function DraggerOver(){ $dragger.css("background-color", "#ccc").css("color", "#666").css("border-left-color", "#fff").css("border-right-color", "#555"); } function DraggerOut(){ $dragger.css("background-color", "#999").css("color", "#666").css("border-left-color", "#ccc").css("border-right-color", "#555"); } } else { //disable scrollbar if content is short $dragger.css("display","none"); $dragger_container.css("display","none"); } }); </script>
Feel free to use it wherever-however you like. Enjoy
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu