form Context highlighting using jQuery
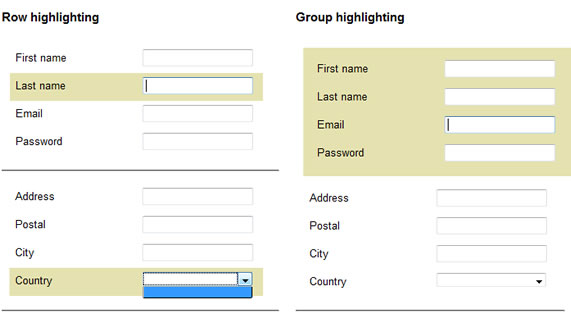
Since the forms were often very complex and had (too much I would say) controls on them, I needed to focus a user's attention on the current context. I first thought to highlight the current row.
The image above shows that whatever user do, current row will be highlighted. The html markup for the example above will look like this:
<h3>Sample web form</h3> <div class="content"> <div class="row"> <div class="left">First name</div> <div class="right"><input name="Text1" type="text" class="text" /></div> <div class="clear"></div> </div> <div class="row"> <div class="left">Last name</div> <div class="right"><input name="Text1" type="text" class="text" /></div> <div class="clear"></div> </div> <div class="row"> <div class="left">Email</div> <div class="right"><input name="Text1" type="text" class="text" /></div> <div class="clear"></div> </div> <div class="row"> <div class="left">Password</div> <div class="right"><input name="Text1" type="text" class="text" /></div> <div class="clear"></div> </div> </div> <hr /> <!- Other rows here --> </div> <input name="Button1" type="button" value="Do some action" />
And CSS classes are pretty simple as well:
body{ font-family:Arial, Helvetica, sans-serif; font-size: 13px; } .content{ padding:10px; width:370px } .left{ width:150px; float:left; padding:7px 0px 0px 7px; min-height:24px; } .right{ width:200px; float:left; padding:5px; min-height:24px; } .clear{ float:none; clear:both; height:0px; } .row{ background-color:none; display:block; min-height:32px; } .text{ width:190px; } .ruler{ width:400px; border-bottom:dashed 1px #dcdcdc; } tr:focus{ background-color:#fcfcf0; } td{ vertical-align:top; } .over{ background-color:#f0f0f0; } .out{ background-color:none; }
Each "row" consists of two div's, one for a label and other for an input field(s). So what we tried to achieve it to highlight a current row whenever user click on any element inside that row. It could be an input field or a label.
Now let's see the simplicity of jQuery:
$(document).ready(function() { $('.left, .content input, .content textarea, .content select').focus(function(){ $(this).parents('.row').addClass("over"); }).blur(function(){ $(this).parents('.row').removeClass("over"); }); });
Whenever an input, textarea, select, or element that has "left" CSS class get focus, append "over" CSS class to all parents that have "row" CSS class. Also, whenever an input, textarea, select, or element that has "left" CSS class lose focus, remove "over" CSS class from all parents that have "row" CSS class.
This was very interesting and extremely simple solution, but in a forms more complex that the one in the example above it just didn't make much improvement. What I wanted actually was to highlight a group of related fields. Thanks to jQuery it was very easy to change the rules:
$(document).ready(function() { $('.left, .content input, .content textarea, .content select').focus(function(){ $(this).parents('.content').addClass("over"); }).blur(function(){ $(this).parents('.content').removeClass("over"); }); });
Note that the only change is parents() target. Instead of changing only parent row, we can highlight a whole group of rows. Each div that has "content" CSS class will be highlighted whenever any of his children get focus and vice versa.
In very complex web forms this enables users to focus only on a current action. By using attractive color schema and jQuery animations, this can be even more interesting.
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu