useful circulate Slider with jQuery
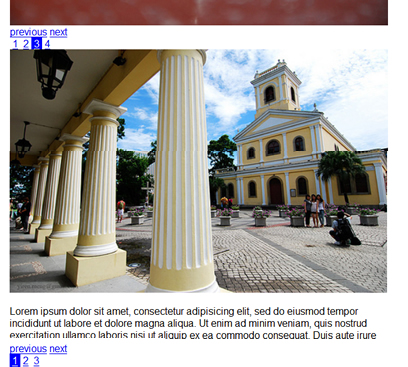
we’re going to use Nathan Searles’ jQuery loopedSlider, a plugin made for jQuery that solves a simple problem, the looping of slide content. It was created to be easy to implement, smooth and most of all end the “content rewind” that most other content sliders suffer from. This is going to be a quick and easy tutorial. You can just copy and paste the code and have yourself a looping slider in minutes.
Markup
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> <title>loopedSlider jQuery Plugin</title> <script src="http://jqueryjs.googlecode.com/files/jquery-1.3.2.min.js" type="text/javascript" charset="utf-8"></script> <script src="loopedslider.js" type="text/javascript" charset="utf-8"></script> <style type="text/css" media="screen"> </style> </head> <body> <div id="loopedSlider"> <div class="container"> <div class="slides"> <div><img src="image-01.jpg" width="600" height="393" alt="First Image" /></div> <div><img src="image-02.jpg" width="600" height="393" alt="Second Image" /></div> <div><img src="image-03.jpg" width="600" height="393" alt="Third Image" /></div> <div><img src="image-04.jpg" width="600" height="393" alt="Fourth Image" /></div> </div> </div> <a href="#" class="previous">previous</a> <a href="#" class="next">next</a> <ul class="pagination"> <li><a href="#">1</a></li> <li><a href="#">2</a></li> <li><a href="#">3</a></li> <li><a href="#">4</a></li> </ul> </div> </body> </html>
Styles (CSS)
/* * Required */ .container { width:600px; height:393px; overflow:hidden; position:relative; cursor:pointer; } .slides { position:absolute; top:0; left:0; } .slides div { position:absolute; top:0; width:600px; display:none; } /* * Optional */ #loopedSlider,#newsSlider { margin:0 auto; width:500px; position:relative; clear:both; } ul.pagination { list-style:none; padding:0; margin:0; } ul.pagination li { float:left; } ul.pagination li a { padding:2px 4px; } ul.pagination li.active a { background:blue; color:white; }
Power Up The Slider
This time we’re going to initialize the plugin from the bottom section of our document in order to load our content first before the calling our plugin.
<script type="text/javascript" charset="utf-8"> $(function(){ $('#loopedSlider').loopedSlider(); }); </script>
Autostart the slider:
<script type="text/javascript" charset="utf-8"> $(function(){ $('#loopedSlider').loopedSlider({ autoStart: 3000 }); }); </script>
Initialize multiple sliders:
<script type="text/javascript" charset="utf-8"> $(function(){ $('#loopedSlider').loopedSlider({ autoStart: 3000 }); $('#anotherSlider').loopedSlider({ autoHeight: 400 }); }); </script>
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu