fancy share box with CSS and jQuery




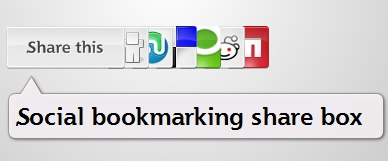
This examples will show you how to turn unordered list (UL) into an fancy social bookmarking sharing box. You will see how to style such box, how to add interactivity, and how to create jQuery plugin that will turn any UL into sharing box.
The idea is to have all of the buttons shown inline and partially hidden. When user hovers over a button it slides to the right and become visible.
Styling unordered list
We will use unordered list to create a simple list that will show icons of popular social bookmarking sites. First list item won't be an icon but rather an image saying "share this".
<ul class="sharebox"> <li><img src="sharethis.png" alt="Share this" /></li> <li><a href="#"><img src="digg_48.png" alt="Sumbit to Digg" /></a></li> <li><a href="#"><img src="sumbleupon_48.png" alt="Sumbit to StumbleUpon" /></a></li> <li><a href="#"><img src="delicious_48.png" alt="Sumbit to Delicious" /></a></li> <li><a href="#"><img src="technorati_48.png" alt="Sumbit to Technorati" /></a></li> <li><a href="#"><img src="reddit_48.png" alt="Sumbit to Reddit" /></a></li> <li><a href="#"><img src="mixx_48.png" alt="Sumbit to Mixx" /></a></li> </ul>
We'll leave HTML anchors empty here so you have to replace them with real links. Now let's add some styles to make this list a little bit nicer.
ul.sharebox { margin:0px; padding:0px; list-style:none; } ul.sharebox li { float:left; margin:0 0 0 0px; padding:0px; } ul.sharebox li a { margin:0 0 0 -24px; display:block; } ul.sharebox li a:hover { margin:0 0 0 -8px; } ul.sharebox li img { border:none;}
Now the list looks fine, but this is not what we wanted to do. Right side of each button (except the last one) is hidden so they won't slide to the right. Each button should be shown under the previous one, and that is not the case here.
To carry out what we wanted from the beginning, we need to involve absolute inside relative positioning. Each list item will be absolutely positioned inside relatively positioned unordered list. This means that each button will appear at position top:0px and left:0px.
ul.sharebox { margin:0px; padding:0px; list-style:none; position:relative;} ul.sharebox li { float:left; margin:0 0 0 0px; padding:0px; position:absolute; }
Adding jQuery
Image above shows how buttons will appear when we make them absolutely positioned. What we have to do next is to involve some jQuery. To make each button appear under the previous one, we have to apply z-index CSS attribute properly and to move each button to its place.
$(document).ready(function(){ var i = 10; var j = 0; $("ul#sharebox li").each(function() { $(this).css("z-index", i) if (j > 0) $(this).css("left", j * 24 + 100 + "px"); i = i - 1; j = j + 1; }); });
The code above iterates through collection of list items and applies z-index to each list item. Z-index starts with 10 and decrement by one for each list item. This means that each button will have z-index less than its predecessor and will appear under it. This is what we wanted from the beginning. This code also assigns values to "left" attribute for each list item. Each list item will be partially shown, exactly 24px to the right (half of total button width). Also, each button will be moved 100px to the right because that space is reserved for "share this" image. This is why we don't set "left" attribute to the first list item (j>0).
Creating plugin
This can be turned into plugin easily. Create sharebox.js file, and copy&paste jQuery function we created previously. Instead of "ul#sharebox li" selector, we'll use local variable "element" that refers to the element to which plugin is applied. We'll then select all list items using find("li") function. Everything else remains the same.
(function($){ $.fn.sharebox = function(){ var element = this; var i = 10; var j = 0; $(element).find("li").each(function(){ $(this).css("z-index", i) if (j>0) $(this).css("left", j * 24 + 100 + "px"); i = i - 1; j = j + 1; }); } })(jQuery);
To apply plugin to some unordered list you just type this:
$("#list").sharebox();
Where "list" is id of unordered list.
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu