Twitter style Ajax Sign In Form with jQuery and CSS3
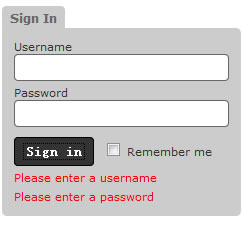
This is a Twitter style drop down sign in form that uses jQuery and Ajax. The form sends an Ajax request to a server script to verify the username and password. The script also includes JavaScript validation. This script is dependent on the jQuery Form Plugin.
The Markup
First, we'll place the page content inside a container. This example checks an ASP session value to determine if the user has already logged in and shows the appropriate button. The 'href' value for the 'Sign In' button is used in case JavaScript is turned off in the user's browser. The form's 'action' value entered here is the URL where the Ajax request is sent. It's important that you change this to your own server side page containing your login routine.
<div id="container"> <div id="signbtn"> <% If Session("loggedin") Then %> <a href="logout.asp" class="btnsignout">Sign Out</a> <% Else %> <a href="signin.asp" class="btnsignin">Sign In</a> <% End If %> </div> <div id="frmsignin"> <form method="post" id="signin" action="test.asp"> <p id="puser"> <label for="username">Username</label><br /> <input id="username" name="username" value="" title="username" tabindex="1" type="text" /> </p> <p> <label for="password">Password</label><br /> <input id="password" name="password" value="" title="password" tabindex="2" type="password" /> </p> <p class="submit"> <input id="submitbtn" value="Sign in" tabindex="3" type="submit" /> <input id ="remember" name="remember" value="1" tabindex="4" type="checkbox" /> <label for="remember">Remember me</label> </p> </form> <p id="msg"></p> </div> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam lorem purus, volutpat nec faucibus vitae, elementum bibendum eros. Pellentesque auctor tristique justo, vel ornare nibh semper ac. Integer adipiscing leo in quam hendrerit faucibus. Vestibulum id rutrum ligula. Integer tristique ligula a diam facilisis vitae rutrum massa egestas. Pellentesque imperdiet, lectus pulvinar egestas pharetra, dolor mi condimentum dolor, ac pulvinar sapien eros nec massa.</p> </div>
The CSS
Here are the styles for the container, signin button, and the form:
body{ padding:20px; font:12px Verdana, Geneva, sans-serif; color:#333; } #container { width:700px; margin:0 auto; padding:13px 10px; border:1px solid #999; } a.btnsignin, a.btnsignout { background:#999; padding:5px 8px; color:#fff; text-decoration:none; font-weight:bold; -webkit-border-radius:4px; -moz-border-radius:4px; border-radius:4px; } a.btnsignin:hover, a.btnsignout:hover { background:#666; } a.btnsigninon { background:#ccc!important; color:#666!important; outline:none; } #frmsignin { display:none; background-color:#ccc; position:absolute; top: 59px; width:215px; padding:12px; *margin-top: 5px; font-size:11px; -moz-border-radius:5px; -moz-border-radius-topleft:0; -webkit-border-radius:5px; -webkit-border-top-left-radius:0; border-radius:5px; border-top-left-radius:0; z-index:100; } #frmsignin input[type=text], #frmsignin input[type=password] { display:block; -moz-border-radius:4px; -webkit-border-radius:4px; border-radius:4px; border:1px solid #666; margin:0 0 5px; padding:5px; width:203px; } #frmsignin p { margin:0; } #frmsignin label { font-weight:normal; } #submitbtn { -moz-border-radius:4px; -webkit-border-radius:4px; border-radius:4px; background-color:#333; border:1px solid #fff; color:#fff; padding:5px 8px; margin:0 5px 0 0; font-weight:bold; } #submitbtn:hover, #submitbtn:focus { border:1px solid #000; cursor:pointer; } .submit { padding-top:5px; } #msg { color:#F00; } #msg img { margin-bottom:-3px; } #msg p { margin:5px 0; } #msg p:last-child { margin-bottom:0px; }
The JavsScript
jQuery and the jQuery Form Plugin are required. Add the following inside your <head> tag:
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script> <script type="text/javascript" src="jquery.form-2.4.0.min.js"></script> <script type="text/javascript" src="jqeasy.dropdown.js"></script>
The dropdown.js file contains a function which looks for a return string value of 'OK' from your server side login routine. If it doesn't receive 'OK' then it displays the error message that you define in your login routine. There are more details in the download below.
The dropdown.js file also contains form validation. Username must be 3 - 20 characters (a-z, 0-9, _) and Password must be 6 - 20 characters (a-z, 0-9, !, @, #, $, %, &, *, (, ), _). Of course, you can edit this validation code based on your own requirements.
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu