Changing Form Input Styles on Focus with jQuery
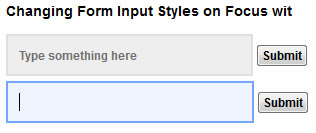
A lot of forms can be boring and plain, don’t let yours blend in. This tutorial will show you how to spice them up with CSS classes and default values that change according to which form item is selected. All with just a splash of jQuery.
Step 1 – The Groundwork
First we’ll need to set up the form we will be using.
<form> <input name="status" id="status" value="Type something here" type="text"/> <input value="Submit" type="submit"/> </form>
I have included “Type something here” as the default value for the input. This is the text that will display initially but disappear when the field gets focus.
Step 2 – The CSS
Now we make the fields look pretty. I’ve done some styling based on my taste, but you will need to include classes for the active/inactive styles of the fields, I have used idleField and focusField.
<style type="text/css"> *{ margin:0; padding:0; font:bold 12px "Lucida Grande", Arial, sans-serif; } body { padding: 10px; } #status{ width:50%; padding:10px; outline:none; height:36px; } .focusField{ border:solid 2px #73A6FF; background:#EFF5FF; color:#000; } .idleField{ background:#EEE; color: #6F6F6F; border: solid 2px #DFDFDF; } </style>
It is also important to note that if you want to remove the blue glow that Safari applies around the field in focus, you will need to include outline:none on those inputs.
Step 3 – Bring on the jQuery
Here’s the part where we add the on focus effects to the inputs in the form. We want a two things to happen when a input field gets focus, 1. The selected field will shift from the inactive class to the active one and 2. The default text inside the box (‘Type something here”) will disappear. Similarly, we want the opposite to happen when the field loses focus.
$(document).ready(function() { $('input[type="text"]').addClass("idleField"); $('input[type="text"]').focus(function() { $(this).removeClass("idleField").addClass("focusField"); if (this.value == this.defaultValue){ this.value = ''; } if(this.value != this.defaultValue){ this.select(); } }); $('input[type="text"]').blur(function() { $(this).removeClass("focusField").addClass("idleField"); if ($.trim(this.value == '')){ this.value = (this.defaultValue ? this.defaultValue : ''); } }); });
Let’s break down what we just put together, initiated by the page loading:
For every text input apply the class idleField to it
$('input[type="text"]').addClass("idleField");
When a text input gets focus, remove the inactive style and add the active style.
$('input[type="text"]').focus(function() { $(this).removeClass("idleField").addClass("focusField"); });
When the user clicks the input field we want the text “Type something here” to go away, if the user has not already typed something else in. The following checks to see if the text value is what it started as when the form loaded. If it is, it gets cleared to make room for user input.
if (this.value == this.defaultValue){ this.value = ''; }
If the user has already typed something in a field, instead of getting cleared when they click on that field again, we want the content to be highlighted.
if(this.value != this.defaultValue){ this.select(); }
The next part of the script handles what happens when an input field loses focus, essentially doing the opposite of what was just explained.
$('input[type="text"]').blur(function() { $(this).removeClass("focusField").addClass("idleField"); if ($.trim(this.value) == ''){ this.value = (this.defaultValue ? this.defaultValue : ''); } });
One difference worth noting is the use of $.trim(), which cleans out white space leading and following user input. Keep in mind that if a user submits the form without altering the default values(“Type something here”), those are the items processed by the form. You can correct this by adding a check/action when the submit button is pressed.
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu